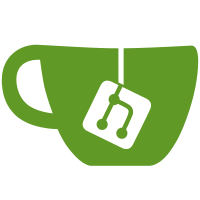
modified: src/bootloader/bootloader.asm new file: src/include/kernel.h modified: src/include/libc.h modified: src/include/paging.h new file: src/include/panic.h modified: src/kernel/kernel.c modified: src/kernel/libc.c modified: src/kernel/page.c new file: src/kernel/panic.c modified: src/link.ld modified: src/makefile modified: tools/page/page.py
46 lines
1.3 KiB
Python
Executable File
46 lines
1.3 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
from math import ceil as c
|
|
def get_offsets(addr):
|
|
if addr % 4096:
|
|
print("You must align to 4096!")
|
|
return 0
|
|
if addr > 2 ** 47:
|
|
print("Address too big!")
|
|
return 0
|
|
|
|
|
|
pte = (addr >> 12) & 0x1ff
|
|
pde = (addr >> 21) & 0x1ff
|
|
pdpe =(addr >> 30) & 0x1ff
|
|
pml4 =(addr >> 39) & 0x1ff
|
|
print("pte:\t{}\npde:\t{}\npdpe:\t{}\npml4:\t{}".format(pte, pde, pdpe, pml4))
|
|
|
|
def get_table_size(addr):
|
|
pte_cnt = c(c(addr / (1 << 12)) / 512) * 512
|
|
pde_cnt = c(c(addr / (1 << 21)) / 512) * 512
|
|
pdpe_cnt = c(c(addr / (1 << 30)) / 512) * 512
|
|
pml4_cnt = c(c(addr / (1 << 39)) / 512) * 512
|
|
|
|
|
|
return((pte_cnt + pde_cnt + pdpe_cnt + pml4_cnt) * 8)
|
|
|
|
def get_palloc_table_size(tb, base, size):
|
|
tsize = 24
|
|
for x in range(8):
|
|
bs = tsize
|
|
tsize += c(((size / (0x1000 * (1 << x))) / 64)) * 8
|
|
print(tsize - bs)
|
|
print("buddy: {} - {} > {}".format(
|
|
hex(bs + tb),
|
|
hex((tsize + tb) - 1),
|
|
(size / (0x1000 * (1 << x))) / 64
|
|
))
|
|
return(tsize)
|
|
|
|
# tablebase, base, size
|
|
free_chunks = [[0x200000, 0x100000, 3220041728], [0x22fce0, 0x100000000, 1073741824]]
|
|
|
|
ts = get_palloc_table_size(free_chunks[0][0], free_chunks[0][1], free_chunks[0][2])
|
|
print(hex(ts))
|
|
|